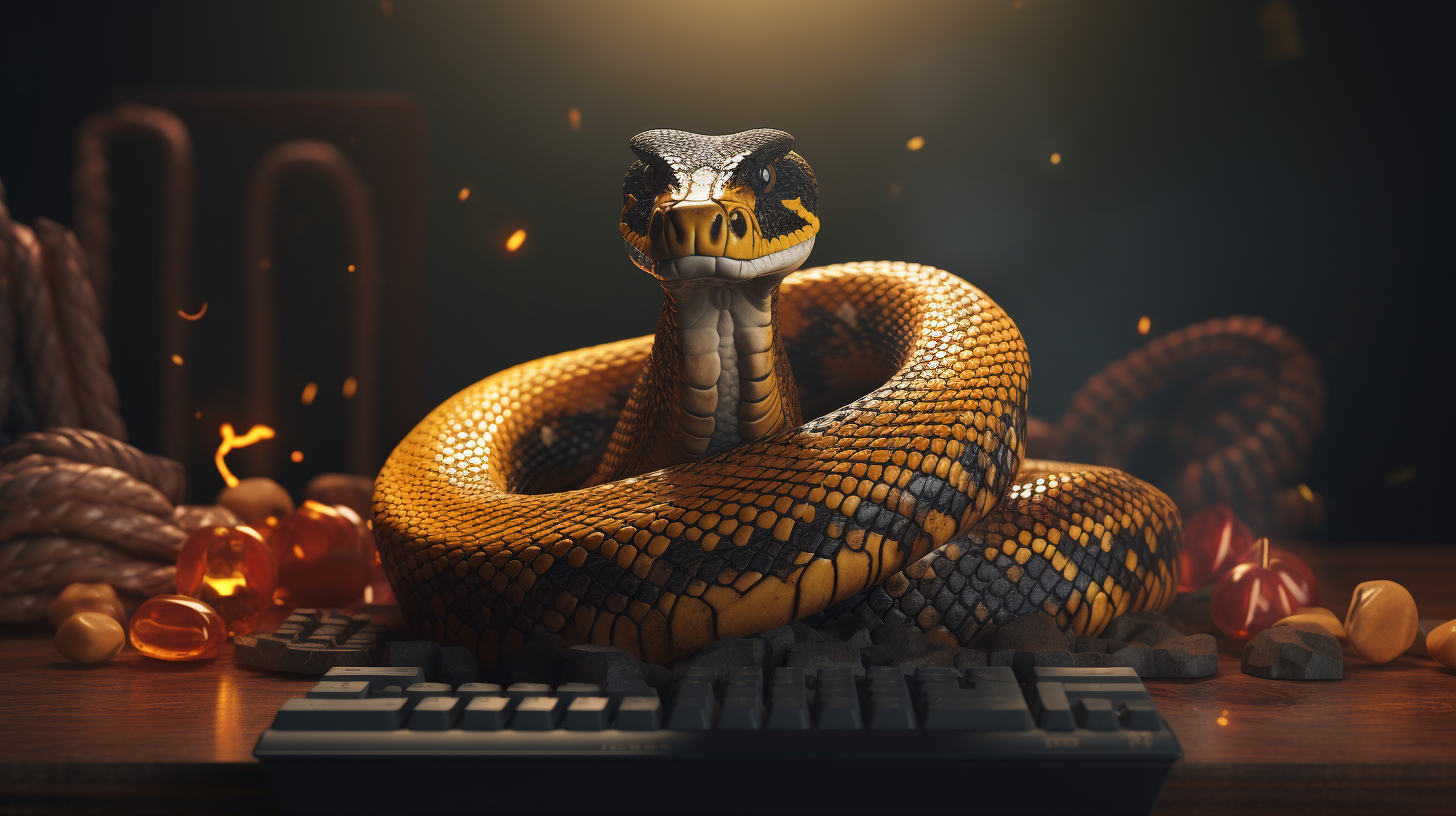
Python is a programming language that has become very popular in application development. We will continue to progress by looking at the concept of abstract classes in Python and how to manipulate them.
Previous tutorial: #11 – Inheritance
This tutorial is also available in video format:
Abstract Classes An abstract class in object-oriented programming (OOP) is a class that cannot be instantiated itself and serves as a model for other classes. It can contain abstract methods, which are methods that have no implementation in the abstract class and must be implemented by the child classes.
Here are some key features of abstract classes:
- Not Instantiable: You cannot create objects directly from an abstract class. It serves as a model for other classes.
- Abstract Methods: An abstract class can contain abstract methods. These methods have no definition in the abstract class, but all derived classes must implement them. This allows defining a common interface for multiple classes.
- Inheritance: Classes that inherit from an abstract class must provide implementations for all abstract methods of the parent class.
Example:
from abc import ABC
class Food(ABC):
name = ''
def showName():
print('Food')
banana = Food('banana')
banana.showName()
To create an abstract class in Python, you need to import a new module. To include an additional module, write at the beginning of the program: from abc import ABC
.
For the class to benefit from this abstraction, it must inherit the class to ABC, hence the line Food(ABC):
.
However, since the class loses its instance notion, the methods of the abstract class can no longer pass the self
, which is my own instance by default. This is why we removed it in def showName():
. This is true because we are trying to create an object from the Food class.
However, in this state, it is quite limited because we can indeed instantiate the method of the parent class if it is overridden by the child class. You will then need to indicate self
as the first parameter because it will be called from an instantiated class.
from abc import ABC
class Food(ABC):
name = ''
def showName(self):
print(self.name)
class Fruit(Food):
isFruity = ''
def __init__(self, name, isFruity):
self.name = name
self.isFruity = isFruity
def showName(self):
super().showName()
banana = Fruit('banana', 'yes')
banana.showName()
Some will tell you that for readability reasons, it is preferable to create an intermediate class and leave the abstract class entirely uninstantiated. I’ll let you make up your own mind on the subject.
However, Python with the abstractmethod
module also allows defining abstract methods, i.e., uninstantiated methods. Abstract methods cannot receive the self
parameter by default, which represents an instance.
So, we imported a new abstractmethod
module, added the @abstractmethod
annotation (this is called an annotation) to apply this abstraction to the showName
method. This then requires all child classes to implement the showName(self)
method.
from abc import ABC, abstractmethod
class Food(ABC):
name = ''
def showName(self):
pass
class Fruit(Food):
isFruity = ''
def __init__(self, name, isFruity):
self.name = name
self.isFruity = isFruity
def showName(self):
print(self.name)
banana = Fruit('banana', 'yes')
banana.showName()
This is very practical to impose a minimum model on child classes that will always have to implement the methods defined in abstraction in the abstract class. This helps avoid development errors when dealing with increasingly complex programs.
Abstract classes used in this way become imposed models.
Conclusion
We have seen new useful concepts about objects in Python that are essential to know to create quality programs. We will go further in the next chapter of our learning about Python, which offers many more secrets.
Next chapter:#13 – Object Typing
Be the first to comment