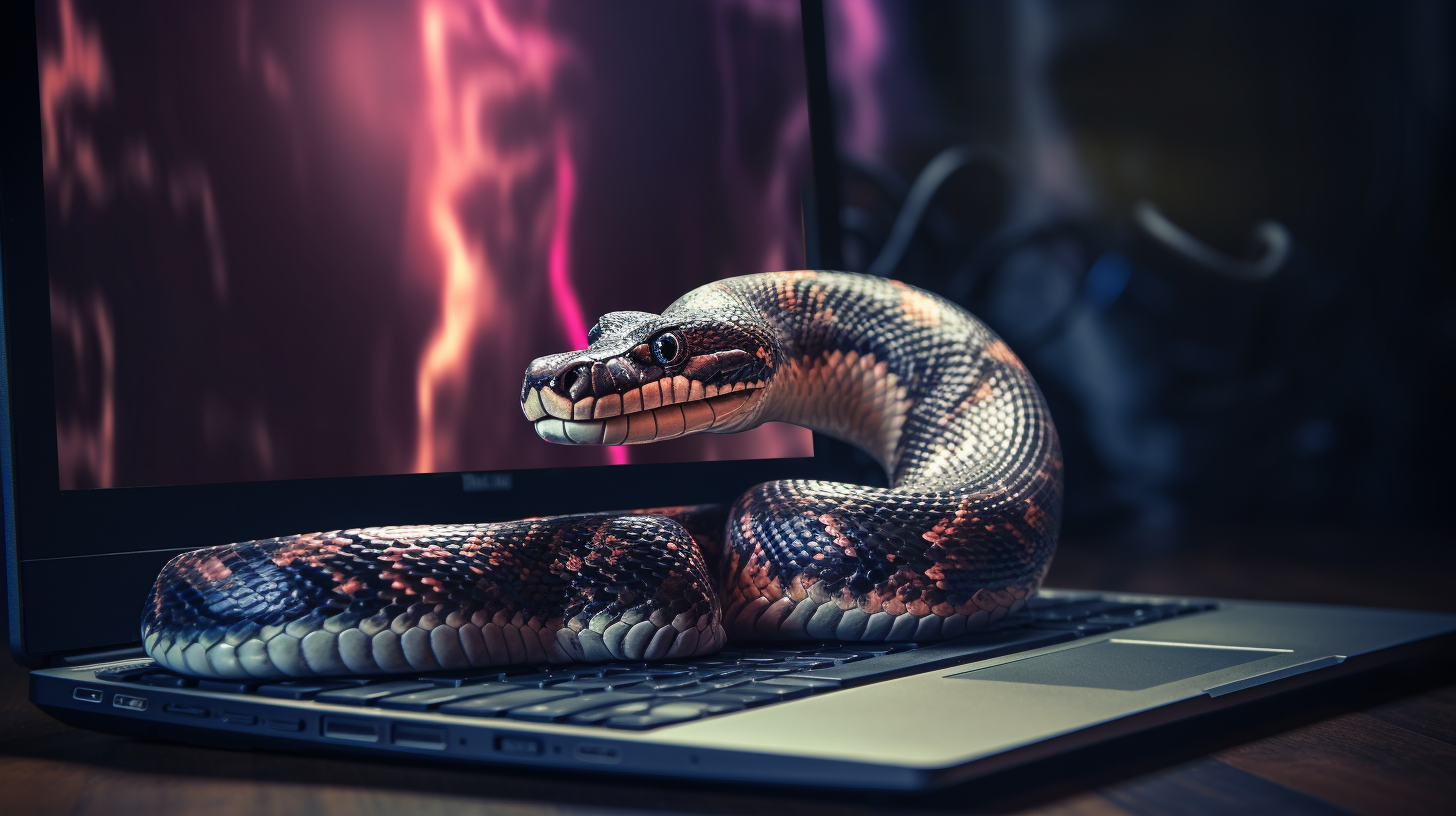
Python has become a highly popular programming language for application development. In this tutorial, we will continue our exploration by delving into the concept of object typing in Python and how to manipulate it.
Previous tutorial: #12 – Abstract Classes
Object Typing
In Python, it is possible to perform object typing to make our objects more secure in their usage. When we talk about “typing,” we mean enforcing the expected variable type for input and output of a method.
To provide an indication without causing an error if the wrong type is specified, the following nomenclature should be used:
variable: type
to type input parameters-> type
for method return types
Here’s an example:
class Fruit():
nom = ''
def __init__ (self, nom: str):
self.nom = nom
def retourneNom(self) -> str:
return self.nom
banane = Fruit('banane')
print(banane.retourneNom())
To make type indications strict, you can use a strict-hint package, which needs to be installed manually in some environments. Afterward, in your program, import your package with the line from strict_hint import strict
and annotate the methods with @strict
where you want to enforce typing.
from strict_hint import strict
class Fruit():
nom = ''
def __init__ (self, nom: str):
self.nom = nom
def retourneNom(self) -> str:
return self.nom
banane = Fruit('banane')
print(banane.retourneNom())
While str
enforces the passing of a string, you can also mandate integers with int
, decimals with float
, booleans with bool
, and even lists or dictionaries. It is also possible to enforce passing an object by the name of its class.
Here’s an example where we must pass an object created from the Color class:
from strict_hint import strict
class Color():
nom = ''
class Fruit():
nom = ''
def __init__ (self, nom: str, couleur: Color):
self.nom = nom
self.color = color
def retourneNom(self) -> str:
return self.nom
yellow = Color()
banana = Fruit('banana', yellow)
print(banana.retourneNom())
Conclusion
We’ve explored valuable new concepts about objects in Python that are essential to know for creating quality programs. In the next chapter of our Python learning journey, we will delve even deeper into the language, uncovering more of its secrets.
Next chapter : #14 – File Manipulation
Be the first to comment