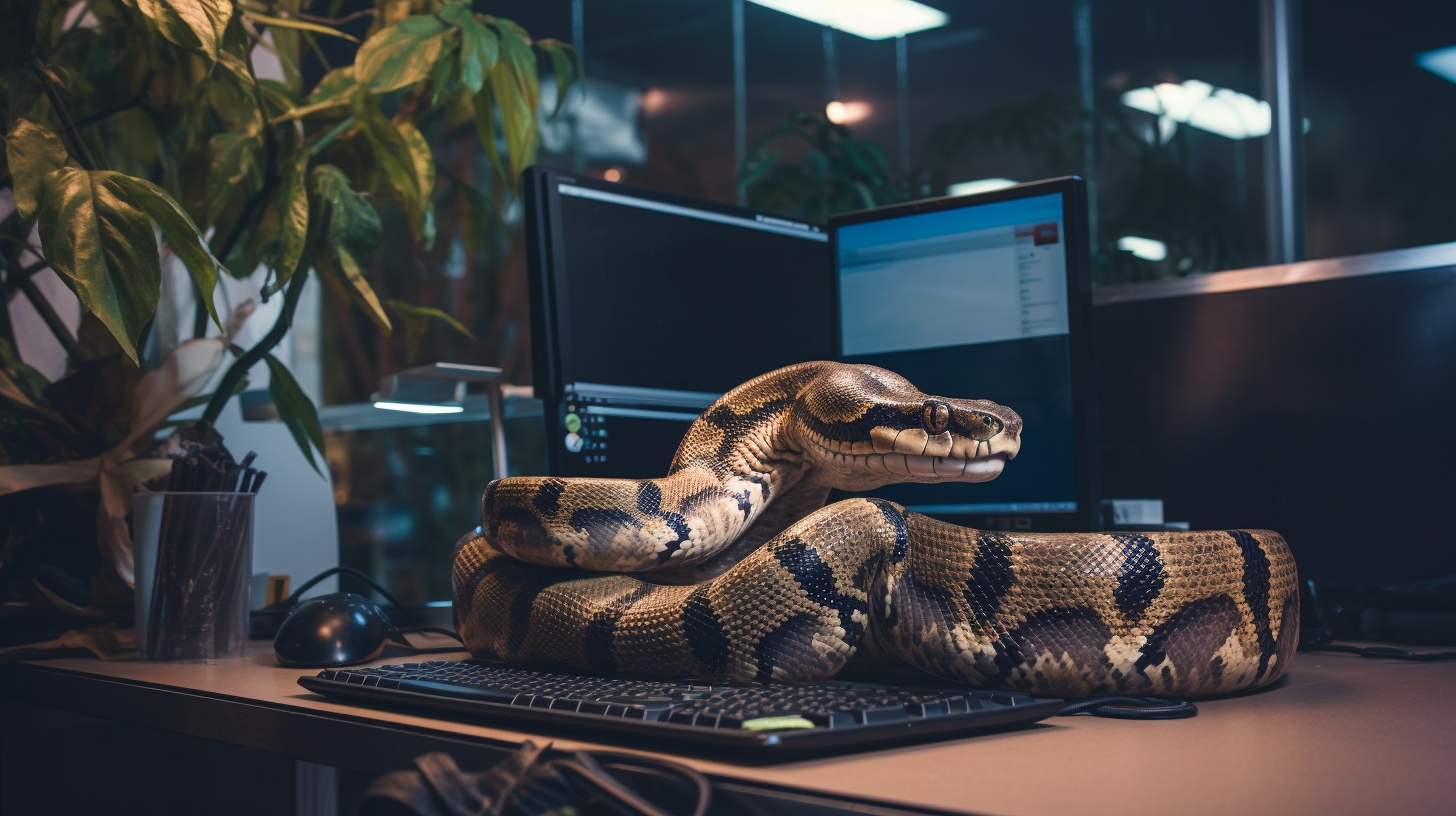
Advanced Object Concepts – Python is a development language that has become very popular in application development. We will continue to move forward by looking at advanced notions about objects in python and how to manipulate them.
Previous tutorial: Tutorial Python – Classes and Objects (9)
Advanced Object Concepts
Python, a language that has become very popular in application development, offers advanced features for working with objects. In this second chapter on the topic, we will explore new useful concepts.
Advanced Parameter Handling
When defining parameters, it is possible, during a call, to precisely target them by specifying the parameter name. This is entirely possible with a regular function.
class Fruit: nom = '' def __init__(self, nom): self.nom = nom banane = Fruit(nom='banane')
Even though “nom” is the first parameter to provide here, we can specifically target it. While it might not make much sense in this context beyond improving readability, here’s an example that illustrates the possibilities:
class Fruit: nom = '' taille = '' def __init__(self, nom, taille): self.nom = nom banane = Fruit(taille='petite', nom='banane')
Here, we call the parameters in a different order than expected. In some cases, this can be useful, but be cautious not to overuse it.
Like functions, we can provide default parameters to class functions. Here’s how it can be done:
class Fruit: nom = '' taille = '' def __init__(self, taille='petite', nom): self.nom = nom banane = Fruit(nom='banane')
Here, we can omit specifying the “taille” parameter, which already has a default parameter, and only pass the “nom” parameter.
Modifying an Object Parameter
We hadn’t seen it before, but it is entirely possible to modify an object parameter after its creation in this way:
class Fruit: nom = '' taille = '' def __init__(self, taille='petite', nom): self.nom = nom banane = Fruit(nom='banane') banane.taille = 'moyen'
By calling a parameter and using the assignment operator “=”, we can modify the value of the parameter from outside the class.
Creating a New Parameter
Similarly, if the parameter was not defined by default in the class, this allows creating the new parameter from the outside:
class Fruit: nom = '' def __init__(self, nom): self.nom = nom banane = Fruit('banane') banane.taille = 'moyen'
Creating a Parameter Global to Objects of the Same Class
Python allows creating a specific parameter that will be shared by all objects created from the same class. This can be very useful in certain cases.
class Fruit: nom = '' counter = 0 def __init__(self, nom): self.nom = nom self.countObject() @classmethod def countObject(cls): cls.counter += 1 banane = Fruit('banane') fraise = Fruit('fraise') print(banane.counter) print(fraise.counter)
Here, we create a specific function with @classmethod
and by passing a special parameter cls
to impact the parameter across all objects created from this class. With each object creation using the magical __init__
function, we add 1 to the counter
parameter through this specific function we called countObject()
.
Thus, this program will display 2 2, meaning it has indeed impacted the counter
parameter in all objects created from this Fruit class.
Creating Static Methods
Python allows creating static methods, a concept that exists in some object-oriented programming languages. This allows creating a method that runs on the class itself and not on the child instances (objects created from this class). These functions often create utility functions.
If you cannot see the usefulness at the moment, know that it also has an impact on the “memory” used.
Here’s a possible example:
class Fruit: nom = '' def __init__(self, nom): self.nom = nom @staticmethod def message(): print('5 fruits and vegetables a day') banane = Fruit('banane') banane.message()
Conclusion – Objects
We have seen new useful concepts about objects in Python, which are essential to know to create quality programs. We will go further in the next chapter of our learning about Python, which still holds many secrets.
*Next Chapter: Tutorial Python – More Advanced Topics (11)
Be the first to comment