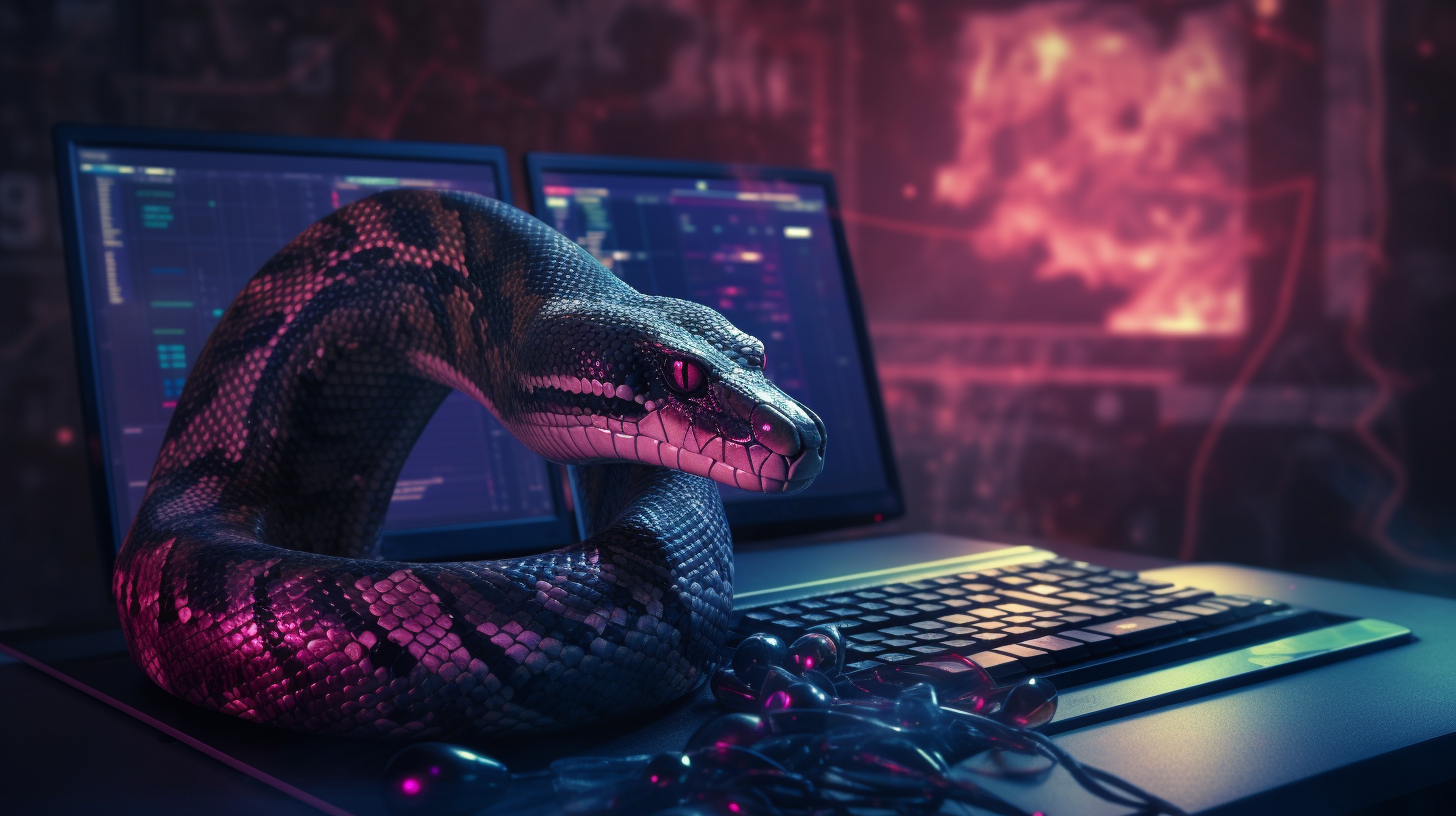
Python With – In Python, the ‘with’ statement is used in exception handling to make the code cleaner and much more readable.
Let’s take advantage of this article to better understand its usage.
You can find all our tutorials on this page: click here
Python With Tutorial
The ‘with’ statement simplifies the management of common resources such as file streams.
Let’s look at the following example of how using the ‘with’ statement makes the code cleaner.
# Writing to a file
# 1) without using the 'with' statement
file = open('file_path', 'w')
file.write('hello world !')
file.close()# 2) without using the 'with' statement
file = open('file_path', 'w')
try:
file.write('hello world')
finally:
file.close()# using the 'with' statement
with
open('file_path', 'w') as file:
file.write('hello world !')
Please note that, unlike the first two approaches, it is not necessary to call file.close()
when using the ‘with’ statement. The ‘with’ statement handles the acquisition and proper release of resources itself. This makes the code more readable and reduces the number of lines by half.
In the first approach, an exception occurring during the file.write()
call might prevent the file from being closed properly, introducing several bugs into the code. In other words, many changes to files will only take effect when the file is properly closed.
The second approach in the above example handles all exceptions, but using the ‘with’ statement makes the code more concise and much more readable. Therefore, using the ‘with’ statement helps prevent errors and leaks by ensuring that a resource is properly released when the code using that resource is fully executed. The ‘with’ statement is commonly used with file streams, as demonstrated above, as well as with locks, sockets, child processes, and Telnet connections, among others.
The ‘with’ Statement in Our Objects
There is nothing extraordinary about the open()
function that makes it usable with the ‘with’ statement, and similar functionality can be implemented in objects that we have defined.
By supporting the ‘with’ statement in your own objects, you ensure that you never leave resources open. To use the ‘with’ statement in custom objects, you simply need to include the __enter__()
and __exit__()
methods in the object’s methods, as shown in the following example for clarity.
# a simple file writer object
class WriteToFile(object):
def __init__(self, file_name):
self.file_name = file_namedef __enter__(self):
self.file = open(self.file_name, 'w')
return self.filedef __exit__(self, *args):
self.file.close()# using 'with' on my WriteToFile object
with
WriteToFile('my_file.txt') as myFile:
myFile.write('hello world')
Now let’s see how Python interprets our code above.
As soon as the execution enters the context of the ‘with’ statement, a WriteToFile
object is created, and Python then calls the __enter__()
method. Inside this __enter__()
method, you perform the initialization of the resource you want to use in the object. It is important to note that this __enter__()
method must always return a handle to the acquired resource.
But what do we mean by “resource handles”? These are handles provided by the operating system to access the requested resources. In the case of the given example, file
is a resource handle for the file stream.
Once the code inside the ‘with’ block has executed, the __exit__()
method is automatically called. It is in this __exit__()
method that all acquired resources are released. This is precisely how we use the ‘with’ statement with user-defined objects.
This interface, based on the __enter__()
and __exit__()
methods, which supports the ‘with’ statement with custom objects, is called a “Context Manager.”
Conclusion – Python With
Now the Python ‘with’ statement has no more secrets for you. Hoping that this little tutorial will be useful to enhance all your Python codes.
Be the first to comment