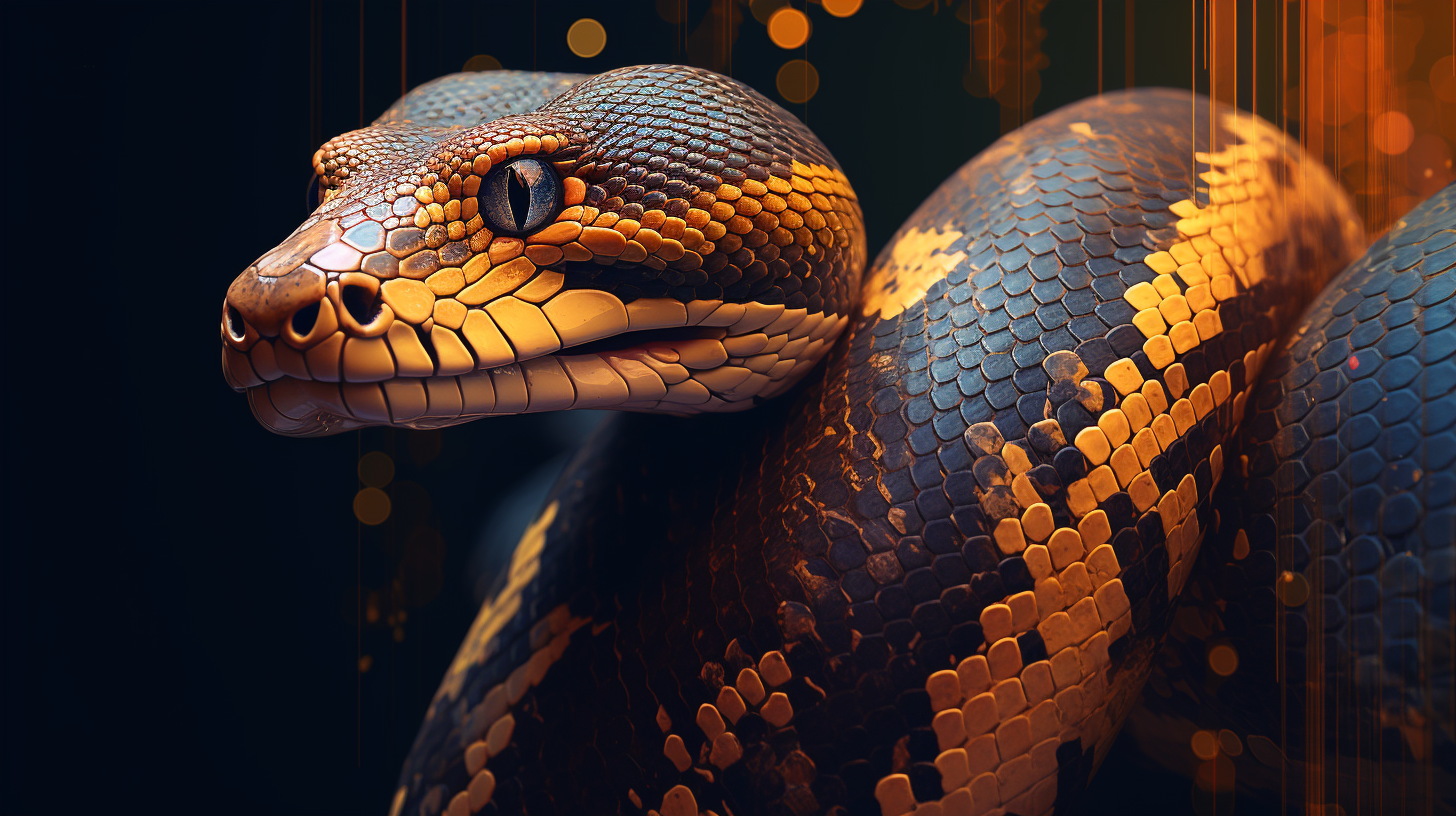
Python has become an immensely popular programming language for application development. In this tutorial, we’ll delve into Python dictionaries and explore how to manipulate them.
Previous tutorial: #6 – Functions
Python Dictionaries
Python dictionaries are advanced lists essential for developing sophisticated applications. Unlike conventional lists, dictionaries allow indexing with strings (text) instead of numerical indices. However, this difference adds numerous possibilities.
Let’s start with a basic example:
print(monId)
monId = {
"nom": "judicael",
"age": 42,
}
This will display our dictionary:
{"judicael", "age": 42}
It’s also possible to display a specific value from our dictionary by targeting an element with its index.
Here’s an example to display “judicael”:
print(monId[“nom”])
monId = {
"nom": "judicael",
"age": 42,
}
Encapsulating a List in a Dictionary
You can certainly place a list within our dictionary for a specific element.
print(monId[“nom”][1])
monId = {
"nom": "judicael",
"age": 42,
"competence": [
"coach",
"dev"
]
}
This would display “dev,” which is index 1 of the list included as the value of the “competence” index in our dictionary.
Multi-level Python Dictionaries
It’s also possible to encapsulate a dictionary as the value of one of the elements of a dictionary. This works in the same way, as shown in the example below:
print(monId[“nom”][“coach”])
monId = {
"nom": "judicael",
"age": 42,
"competence": {
"coach": "agile",
"dev": "python",
}
}
If you’ve grasped the concept, you’ll know that this program will display “agile.”
Modifying an Element in My Dictionary
Obviously, it’s possible to manipulate Python dictionaries. To modify an element in my dictionary, we’ll use the same syntax as modifying an element in a list by targeting the element with its index:
monId[“age”] = 43
monId = {
"nom": "judicael",
"age": 42,
}
print(monId[“age”])
This program will display the new value of the “age” element, which is 43.
Adding an Element to My Dictionary
Similarly, it will be possible to add an element to my dictionary. If I assign a value to an element that doesn’t exist in the dictionary, it will be automatically added.
monId[“taille”] = 180
monId = {
"nom": "judicael",
"age": 42,
}
print(monId[“taille”])
This program will add the “taille” element to the dictionary, which I can then display by calling it in my print function.
It’s possible, in the same way, to add a list or a dictionary instead of this integer variable.
Deleting an Element from My Dictionary
Python also allows deleting an element from the dictionary using the del
keyword, which we had already seen in lists. Its usage is identical, as shown in the example below:
del monId[“age”]
monId = {
"nom": "judicael",
"age": 42,
}
print(monId)
This program will display my dictionary without the deleted “age” element:
{"nom": "judicael"}
Finding an Element by its Value
As with lists, it’s possible to search for the presence of an element by its value using the in
keyword:
monId = {
"nom": "judicael",
"age": 42,
}
print("judicael" in monId)
print("robert" in monId)
This program returns True
and False
because the first value is present in the dictionary, while the second is absent.
Conclusion – Python Dictionaries
We’ve covered quite a bit about Python dictionaries, even though we’ll explore more in later tutorials. We’ll delve deeper in the next chapter of our Python learning journey, which still holds many secrets.
Next Chapter: #8 – Loops
Be the first to comment