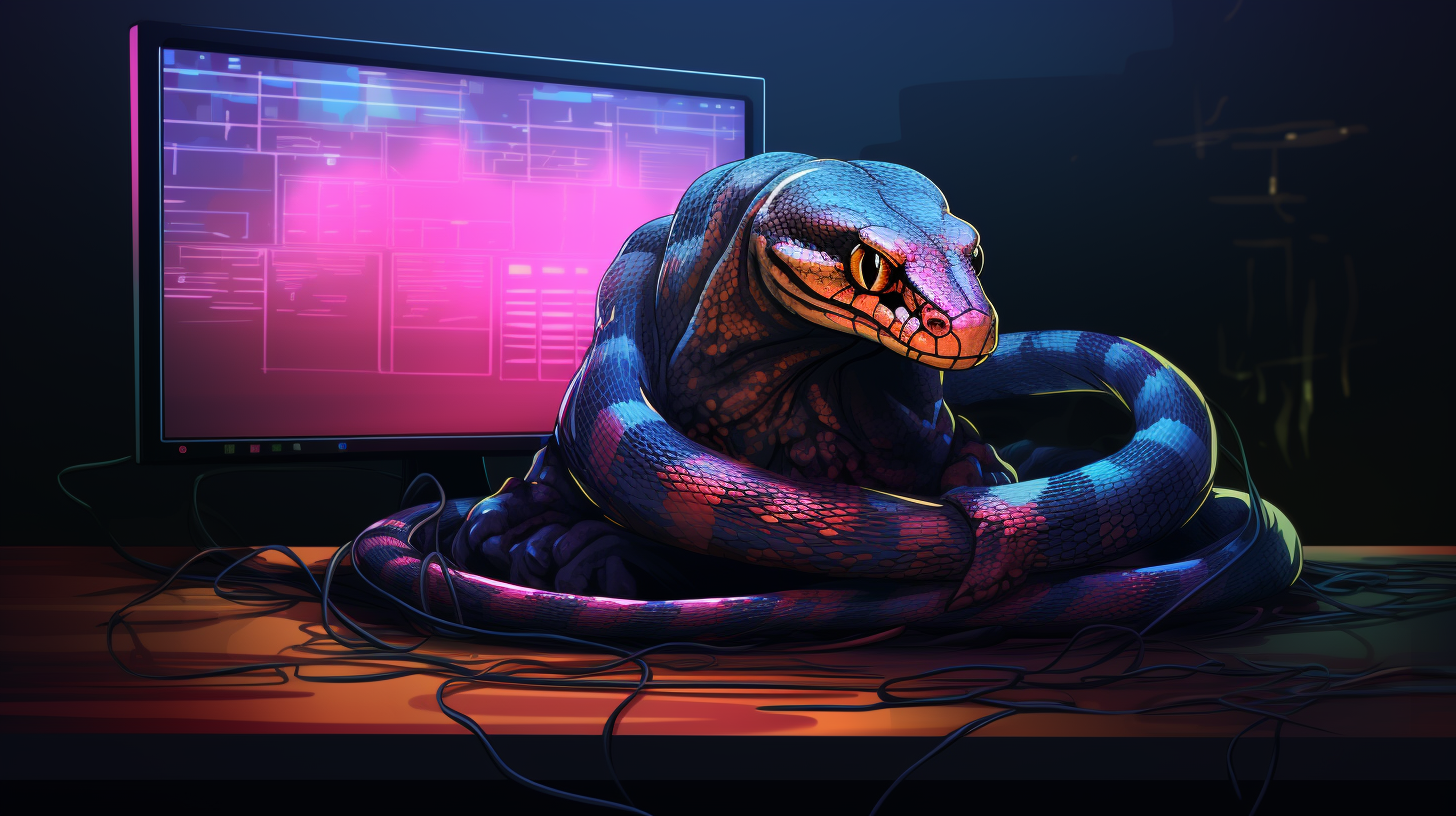
Python Function – Python is a development language that has become very popular in application development. We’ll continue moving forward by looking at python functions and how to manipulate them.
Previous tutorial: Python list tutorial (5)
Python Functions
Functions are essential elements in programming. We’ve already seen built-in functions in the Python language (previously created), but now we’ll learn how to create our own functions to streamline our work. This helps avoid repeatedly writing the same lines of code.
Functions are defined by a name followed by parentheses; inside the parentheses, you can pass parameters.
Here are some examples of built-in functions we’ve seen in previous tutorials:
print("Hello World")
len(user)
del(user[1:3])
Creating Your Own Function
In Python, as in most programming languages, you can create your functions. However, you’ll need to use names different from those of built-in functions.
Here’s a first example of a function:
def display():
i = 2
print(i)
display()
Even though this example is not very useful, we’ve created our function display()
using the def
keyword. The content of the display()
function is indented (has an extra space at the beginning of the line). This indentation tells the program that this is the content of the previous container.
To execute our function, we use display()
, just as we would use print("Hello World")
.
To understand programming better, the def display()
part and its two lines of content are recorded when the program starts but not executed. It’s only when the program calls display()
that it executes the previously recorded function.
If you run our previous program, it will display: 2.
Function Variables
If we run the following program, we’ll get an error:
def display():
i = 2
print(i)
display()print(i)
The print()
function attempts to display the value of the variable i
. However, the variable i
is defined inside the display()
function and is not accessible outside of it.
In fact, a variable defined within a function is only accessible within that function. It has no global scope.
However, a keyword allows us to use a variable defined outside the function: global
. This keyword refers to a variable defined in the main body of our program and can impact it as needed.
Here’s an example to see how to use it:
def display():
global i
print(i)
i = 2
display()
The function will retrieve the value of the variable i
, defined in the main body of the program, and display it. This program will display 2.
A globally defined variable can also be modified within the function, and this will have a global impact.
i = 2
def increment():
global i
i = i + 1
increment()
print(i)
In this case, the function increments the variable i
inside the function and displays 3 outside the function.
Python Functions Can Return a Value
Using the return
keyword, a Python function can return a value to the caller.
j = returnTwo()
def returnTwo():
return 2
print(j)
The function returns the value 2, which is stored in the variable j
and displayed on the screen.
Defining an Argument for the Function
When we used the print("Hello World")
function, we passed an argument, “Hello World,” for the function to display. We can also define one or more parameters in our function.
Our parameter is defined as a new variable directly within the parentheses. This variable can be used directly inside our function.
i = increment(1)
def increment(number):
return number + 1
print(i)
Our program uses the increment
function that we defined, passing the parameter 1
. The function returns 2
, which is then displayed.
We can also define a default value for our parameter like this:
i = increment()
def increment(number=1):
return number + 1
print(i)
With the default parameter variable=value
, if we don’t pass a parameter when calling the increment()
function, it will take the default value.
Without this default value, the program would return an error because it would mean the function expects a value in all cases.
Conclusion – Python Functions
We will continue our Python learning in the next chapter, as this language still holds many secrets. Later on, we’ll explore other key elements related to functions.
Next chapter: Python Dictionaries
Be the first to comment