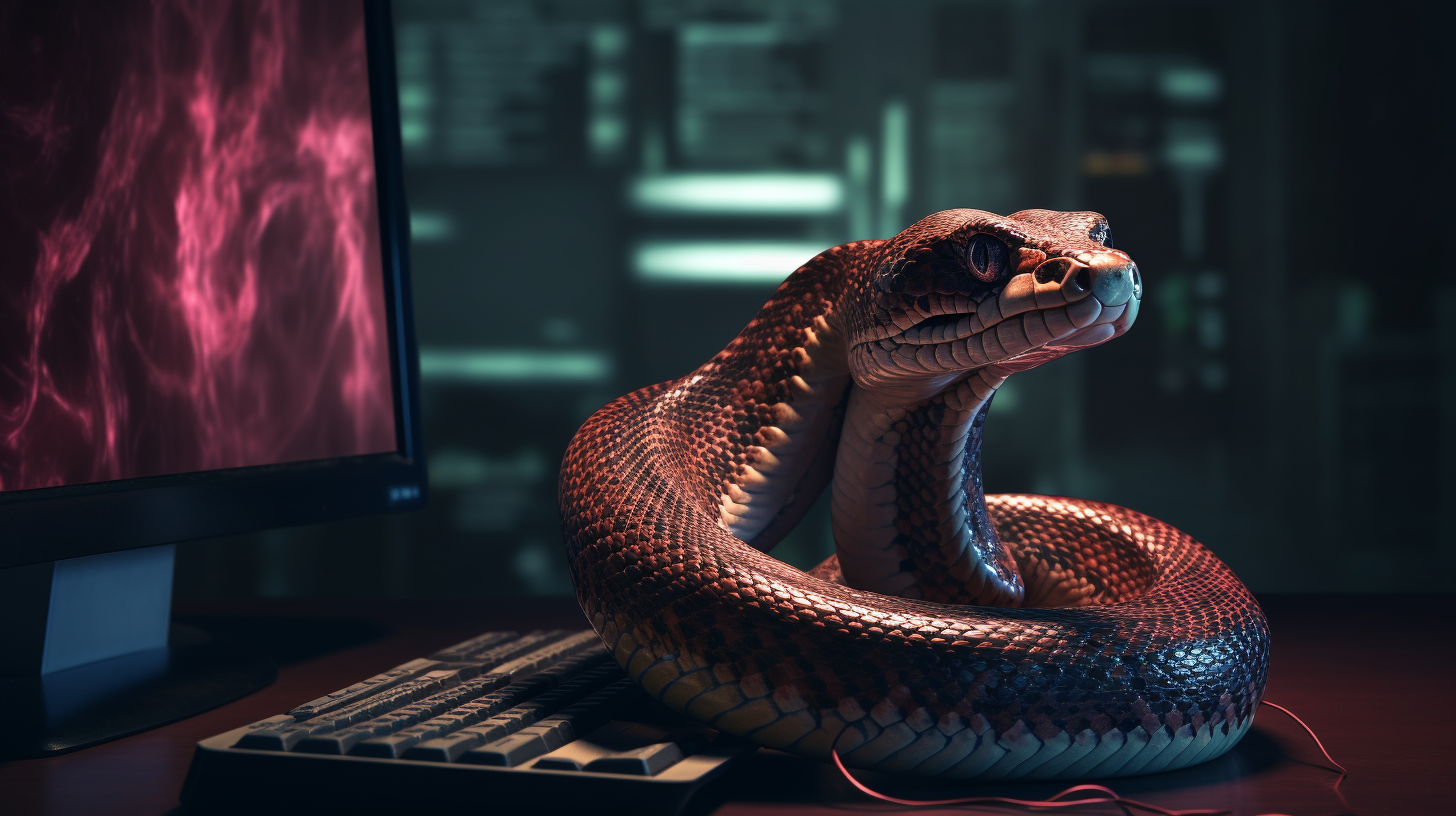
Python has become a very popular development language for application development. We will continue to progress by looking at Python’s if conditions and how to manipulate them.
Previous Tutorial: Python String Tutorial – Strings (3)
Python Conditions
Conditions are essential elements in software development. They allow us to use “if,” “then,” and even “else if”…
Let’s learn by looking at a first example:
myAge = 40 legalAge = 18 myName = "judicael" if myAge > legalAge and myName == "judicael": print("You are of legal age, and your name is Judicael")
We will, of course, take the time to understand everything as there are several concepts that appear in this piece of code.
To begin, we use the “if” keyword to initiate a condition; we are either going to test certain elements to trigger a part of the program if the conditions are met.
The part of the program that will run is the one that is indented (meaning it has more leading space) by one extra tab. You can see that the line containing the print() function starts further than the line containing the “if” statement.
To perform comparisons, we will use comparison operators. Here is the table of those available in Python:
Operator Description
Operator | Description |
> | greater than |
< | less than |
>= | greater than or equal to |
<= | less than or equal to |
== | equal to |
!= | not equal to |
<> | not equal to |
is | is the same object as |
is not | is not not the same object as |
Note that we use “==” to compare equality between two elements because a single “=” corresponds to value assignment.
myAge = 40 legalAge = 18 myName = "judicael" if myAge > legalAge and myName = "nicolas": print("You are of legal age, and your name is Nicolas")
Here, “myName = “nicolas”” doesn’t compare the variable “myName” and the value “nicolas” but assigns the value “nicolas” to the “myName” variable. A successful assignment will return True and validate the condition.
In our example, we also have what’s called a Boolean operator: “and.” This allows you to have multiple conditions to validate the general condition.
There is also the Boolean operator “or,” which means that either the right or left element must be valid to validate the general condition.
myAge = 40 legalAge = 18 myName = "judicael" if myAge > legalAge or myName == "judicael": print("You are of legal age or your name is Judicael")
Rarely used, it is possible to use the Boolean operator to request the opposite, like this: not 8 > 2. This would return False. We won’t go any further into this very particular operator for now.
It is possible to chain conditions to plan different possible cases by using elif (then if) and else. Here is a program that you should understand right away if you have followed everything above.
myAge = 40 legalAge = 18 if myAge > legalAge: print("You are of legal age") elif myAge == legalAge: print("You are just of legal age") else: print("You are a minor")
You can also nest one condition inside another condition, remembering to indent each time, like this:
myAge = 40 legalAge = 18 if myAge >= legalAge: if myAge == legalAge: print("You are just of legal age") else: print("You are of legal age") else: print("You are a minor")
For specific cases, it is possible to write our Python conditions in the form of ternary conditions, which allow you to perform an if… else in a single line. I don’t think this will be useful to you at the beginning of your learning, but I’ll give you an example that you can revisit later:
myAge = 40 legalAge = 18 myText = ("I am a minor", "I am of legal age")[myAge >= legalAge]
Conclusion
We will continue our learning in Python in the next chapter because this language still holds many secrets.
Next Chapter: #5 – Lists
Be the first to comment