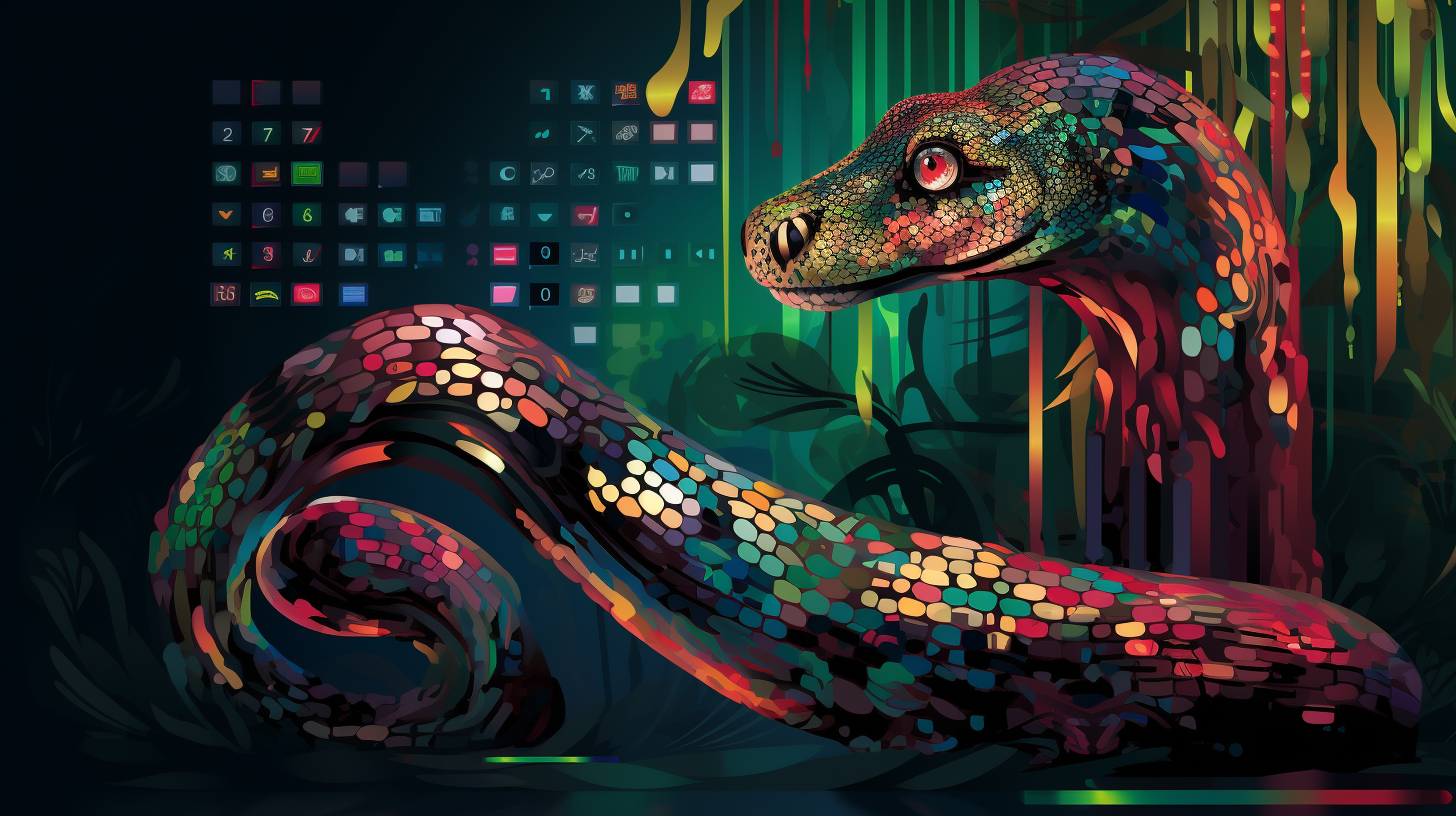
Python is a popular programming language for application development. We’ll continue to explore Python by looking at variables and how to manipulate them.
Previous Tutorial:#1.1 – Commentary
Variables
Definition, Assignment, and Usage
To put it simply, a variable is a container for values. This container can change over time through operations performed on it. It’s also easy to retrieve the value from this container for further use.
A variable is represented by a name, with some mandatory rules, which we’ll discuss later. Let’s begin by understanding how to “assign” a value to a variable we create under the name “myContainer.”
myContainer = 1
Using the print
function as seen in the previous chapter, you can display the value of our container:
myContainer = 1
print(myContainer)
Unlike the previous chapter’s print("Hello World")
, we don’t enclose it in double quotes. Double quotes are used to enclose what we call a fixed string of characters, essentially plain text. If you use print("myContainer")
, it will display “myContainer,” not the value of the variable.
Arithmetic Operators
On numbers, which we will call “integers” or “floats,” we can perform standard mathematical operations using arithmetic operators, presented in the following table:
Description | Caractère |
addition | + |
soustraction | – |
multiplication | * |
division | / |
exponentiel | ** |
division entière | // |
reste de la division entière | % |
Here’s an example to perform an operation within the code we’ve previously written:
myContainer = 1
myContainer = myContainer + 1
print(myContainer)
This will display “2.” To explain, we assigned the value 1 to our variable “myContainer,” then we assigned a new value based on its initial value of 1, adding 1, and finally, we displayed the variable’s value. 1 + 1 = 2, so “myContainer” has a value of 2.
Order of Operations
In our variables, we can apply the concept of order of operations to calculations. Each arithmetic operator has a priority that determines the sequence of operations. For example, when you have the expression “1 + 2 * 3,” the multiplication is performed before addition. Thus, the calculation is performed as follows: 1 + (2 * 3). In terms of hierarchy, the exponentiation operator is evaluated first, followed by the operators *, /, //, and %, and finally, the operators + and -.
When an expression contains multiple operations of the same priority, they are evaluated from left to right. So if you have the expression “1 – 2 – 3,” the calculation occurs as follows: (1 – 2) – 3. In case of confusion, using parentheses explicitly specifies the order of evaluation for arithmetic expressions.
Therefore, you could write (1 + 2) * 3 to define the priority, as if you have some background in mathematics, you know this would change the final result.
Floor Division and Modulus
Two arithmetic operators are particularly suitable for performing calculations with integers: floor division (//) and modulus (%). When an integer D (called the dividend) is divided by another integer d (called the divisor), two results are obtained: a quotient q and a remainder r, such that:
D = q × d + r (where r < d).
The value q is the result of floor division, while the value r is the remainder of the division. For example, when you divide 17 by 5, you get a quotient of 3 and a remainder of 2 because 17 = 3 × 5 + 2.
These two operators are commonly used in various contexts. For example, to determine if an integer is even or odd, you just need to look at the remainder when divided by two. The number is even if the remainder is zero and odd if it’s 1.
Another situation where these operators are useful is in time calculations. If you have a number of seconds and want to convert it into minutes and seconds, you can simply divide by 60. The quotient then represents the number of minutes, while the remainder indicates the remaining seconds. For example, 125 seconds correspond to 125 // 60 = 2 minutes and 125 % 60 = 5 seconds.
Assignment Shortcuts
We had written this previously:
myContainer = 1
myContainer = myContainer + 1
print(myContainer)
Python allows you to speed up the writing of the second line with this:
myContainer = 1
myContainer += 1
print(myContainer)
Writing +=
is exactly the same as writing “myContainer +=”. This allows you to write your code much faster and make it more readable when possible.
Different Variable Types
We’ve seen an “integer” type variable (int), but Python allows you to create other variable types:
- Float (float) is used to represent decimal numbers, including those between 10^-308 and 10^308. The special value
math.inf
is used to represent infinity.
In programming, most development languages distinguish between integers and decimals. It’s important to make the distinction because the results can vary significantly during mathematical operations.
- Complex (complex) is used to represent complex numbers, with the imaginary number denoted as ‘j’. If you’re not familiar with this mathematical concept, it’s not a problem.
- String (str) is a sequence of characters enclosed in either single (‘) or double (“) quotes. Typically, you can use either type of quotation marks interchangeably to delimit a string of characters.
myContainer = "Superman"
print(myContainer)
When you run the program, it will display “Superman.”
There are special characters for creating spaces, line breaks, and more, as shown in the following table:
Description | Caractère |
Display a single backslash \ | \\ |
Display a single quote ‘ | \’ |
Display a double quote “ | \” |
Newline | \n |
Carriage return | \r |
Horizontal tab | \t |
Here’s a simple example (the red is just for demonstration):
myContainer = "Park Lane\nLondon"
print(myContainer)
This code will result in a newline due to \n
:
Park Lane
London
Conclusion – Variables
We’ll delve deeper into variables in the next chapter, as they hold many more secrets to uncover.
Next chapter : Python String Tutorial – String Manipulation
Be the first to comment